Triangulated Surface Series
In This Topic
Triangulated Surface series can display a set of data points with custom X, Y, and Z coordinates as a 3D surface. It is similar to the Mesh Surface series, the main difference being that the Triangulated Surface doesn't require the data points to be ordered in a grid. The control automatically creates a Delaunay triangular network from the input set in order to render the surface. The following image shows a Triangulated Surface chart:
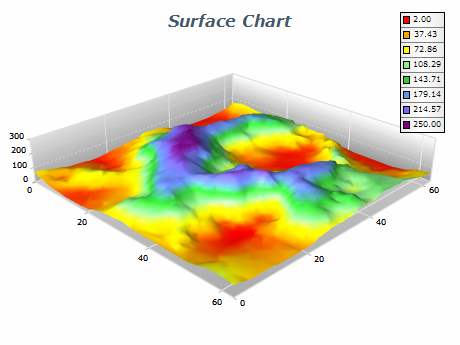
Creating a Triangulated Surface Series
To create a triangulated surface you have to create an instance of the NTriangulatedSurfaceSeries type and add it to the series collection of the chart. Surface series can be displayed only in 3D mode, so it is required to enable 3D for the chart hosting the series. The following code shows how to create a new triangulated surface series and add it to the chart:
C# |
Copy Code
|
// setup chart
NCartesianChart chart = (NCartesianChart)chartView.Surface.Charts[0];
chart.Enable3D = true;
// set aspect 1:1:1
chart.ModelWidth = 50;
chart.ModelHeight = 50;
chart.ModelDepth = 50;
// set projection
chart.Projection.SetPredefinedProjection(ENPredefinedProjection.PerspectiveTilted);
// create a surface series
NTriangulatedSurfaceSeries surface = new NTriangulatedSurfaceSeries();
// add the series to the chart series collection
chart.Series.Add(surface);
|
Triangulated Surface Data
The triangulated surface data is contained in an object of type NTriangulatedSurfaceData which is accessible through the Data property of the NTriangulatedSurfaceSeries class. The data is stored as a one dimensional array of data points, where each data point has X, Y, and Z values, and optionally a color value. The size of the array is controlled with the help of the SetCount method of the NTriangulatedSurfaceData object. The AddValue and SetValue methods can be used to add a new XYZ coordinate, or modify the XYZ coordinate at a specified index. If the X, Y or Z coordinate of the data point are invalid (float.NaN) then the data point will be discarded and it will not take part in the triangulation. You should add at least three distinct points to display a triangulated surface. The code below shows how to create a simple triangulated surface:
C# |
Copy Code
|
// obtain a reference to the Cartesian chart that is created by default
NCartesianChart chart = (NCartesianChart)chartView.Surface.Charts[0];
chart.Enable3D = true;
// set aspect 1:1:1
chart.ModelWidth = 50;
chart.ModelHeight = 50;
chart.ModelDepth = 50;
// set projection
chart.Projection.SetPredefinedProjection(ENPredefinedProjection.PerspectiveTilted);
// create a surface series
NTriangulatedSurfaceSeries surface = new NTriangulatedSurfaceSeries();
chart.Series.Add(surface);
surface.FrameMode = ENSurfaceFrameMode.Mesh;
surface.Name = "Grid Surface";
// point 1
surface .Data.AddValue(3.0, 5.0, 1.4);
// point 2
surface .Data.AddValue(2.0, 1.9, 5.1);
// point 3
surface .Data.AddValue(1, 3.5, 4.7);
|
Triangulated Surface With Custom Colors
When the triangulated surface FillMode is set to ENSurfaceFillMode.CustomColors or FrameColorMode is set to ENSurfaceFrameColorMode.CustomColors the surface will display the filling/frame with custom colors per vertex. In this case, you also need to pass a color value for each vertex. This is achieved by using the SetColor method of the grid surface data object - for example:
C# |
Copy Code
|
surface.Data.UseColors = true;
surface.Data.SetColor(0, Color.Red);
surface.Data.SetColor(1, Color.Green);
surface.Data.SetColor(2, Color.Blue);
|
The following image displays a triangulated surface with custom colors:
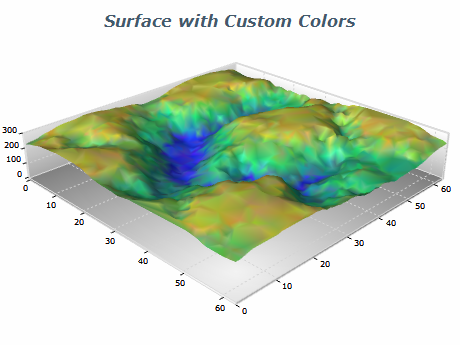
Passing Large Amounts of Data
When you have to feed large amounts of data to a triangulated surface series using the SetValue and SetColor methods will not be very efficient because they result in a function call for each value or color that is set. In such cases, you can consider adding data using unsafe code in which case you directly modify the surface data object in memory. The following code shows how to efficiently add random data points to a triangulated surface series:
C# |
Copy Code
|
// create a surface series
NTriangulatedSurfaceSeries triangulatedSurface= new NTriangulatedSurfaceSeries();
// add the series to the chart series collection
chart.Series.Add(triangulatedSurface);
triangulatedSurface.Data.SetCount(1000);
unsafe
{
fixed (byte* pData = &triangulatedSurface.Data.Data[0])
{
Random random = new Random();
float* pValues = (float*)pData;
int itemSize = triangulatedSurface.Data.DataItemSize;
int count = triangulatedSurface.Data.Count;
// The order of the values is x, y, z. In this case we dynamically modify only x and z.
for (int i = 0; i < count; i++)
{
int dataPointOffset = i * itemSize;
pValues[dataPointOffset] = random.Next(1000);
pValues[dataPointOffset + 1] = random.Next(1000);
pValues[dataPointOffset + 2] = random.Next(1000);
}
triangulatedSurface.Data.OnDataChanged();
}
}
// notify the surface that data has changed
triangulatedSurface.Data.OnDataChanged();
|
See Also